舉報
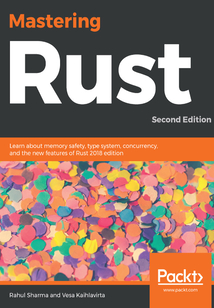
會員
Mastering Rust
Rustisanempoweringlanguagethatprovidesararecombinationofsafety,speed,andzero-costabstractions.MasteringRust–SecondEditionisfilledwithclearandsimpleexplanationsofthelanguagefeaturesalongwithreal-worldexamples,showingyouhowyoucanbuildrobust,scalable,andreliableprograms.ThissecondeditionofthebookimprovesuponthepreviousoneandtouchesonallaspectsthatmakeRustagreatlanguage.WehaveincludedthefeaturesfromlatestRust2018editionsuchasthenewmodulesystem,thesmartercompiler,helpfulerrormessages,andthestableproceduralmacros.You’lllearnhowRustcanbeusedforsystemsprogramming,networkprogramming,andevenontheweb.You’llalsolearntechniquessuchaswritingmemory-safecode,buildingidiomaticRustlibraries,writingefficientasynchronousnetworkingcode,andadvancedmacros.Thebookcontainsamixoftheoryandhands-ontaskssoyouacquiretheskillsaswellastheknowledge,anditalsoprovidesexercisestohammertheconceptsin.Afterreadingthisbook,youwillbeabletoimplementRustforyourenterpriseprojects,writebettertestsanddocumentation,designforperformance,andwriteidiomaticRustcode.
最新章節
- Leave a review - let other readers know what you think
- Other Books You May Enjoy
- Summary
- RR debugger – a quick overview
- Debugger integration with Visual Studio Code
- The gdb basics
品牌:中圖公司
上架時間:2021-07-02 12:29:08
出版社:Packt Publishing
本書數字版權由中圖公司提供,并由其授權上海閱文信息技術有限公司制作發行
- Leave a review - let other readers know what you think 更新時間:2021-07-02 13:36:18
- Other Books You May Enjoy
- Summary
- RR debugger – a quick overview
- Debugger integration with Visual Studio Code
- The gdb basics
- A sample program – buggie
- Setting up gdb
- Prerequisites for debugging
- Debuggers in general
- Introduction to debugging
- Debugging
- Summary
- Other emerging GUI frameworks
- Exercise
- Building a hacker news app using gtk-rs
- GTK+ framework
- Introduction to GUI development
- Building Desktop Applications with Rust
- Summary
- Other languages
- Rust
- Other WebAssembly projects
- Wasm-bindgen
- Rust and WebAssembly
- Ways to generate WebAssembly
- Trying it out online
- Getting started with WebAssembly
- Design goals of WebAssembly
- What is WebAssembly?
- Rust on the Web with WebAssembly
- Summary
- Postgres and the diesel ORM
- Connection pooling with r2d2
- PostgreSQL
- SQLite
- Why do we need data persistence?
- Interacting with Databases in Rust
- Summary
- Building a bookmarks API using Actix-web
- Actix-web basics
- Web frameworks
- hyper as a client – building a URL shortener client
- Hyper server APIs – building a URL shortener
- Typed HTTP with Hyper
- Web applications in Rust
- Building Web Applications with Rust
- Summary
- Building an asynchronous redis server
- Tokio
- Futures
- Mio
- Async abstractions in Rust
- Asynchronous network I/O
- Building a synchronous redis server
- Synchronous network I/O
- Network programming prelude
- Network Programming in Rust
- Summary
- Structured logging using slog
- log4rs
- The env_logger
- log – Rust's logging facade
- Logging in Rust
- Structured logging
- Unstructured logging
- Approaches to logging
- Logging frameworks and their key features
- The need for logging frameworks
- What is logging and why do we need it?
- Logging
- Summary
- Creating native extensions in Rust for Node.js
- Creating native Python extensions with PyO3
- Using external C/C++ libraries from Rust
- Calling Rust code from C
- Calling C code from Rust
- Unsafe traits and implementations
- Unsafe functions and blocks
- What is safe and unsafe really?
- Unsafe Rust and Foreign Function Interfaces
- Summary
- Useful procedural macro crates
- Debugging macros
- Derive macros
- Procedural macros
- Exercises
- Macro use case – writing tests
- A more involved macro – writing a DSL for HashMap initialization
- Repetitions in macros
- macro_rules! token types
- Built-in macros in the standard library
- Creating your first macro with macro_rules!
- Types of macros
- Macros in Rust and their types
- When to use and not use Rust macros
- What is metaprogramming?
- Metaprogramming with Macros
- Summary
- Other crates
- Concurrency using the actor model
- Sync
- Send
- Traits for thread-safety
- What is thread-safety?
- thread-safety in Rust
- Synchronous channels
- Asynchronous channels
- Communicating through message passing
- RwLock
- Shared mutability with Arc and Mutex
- Mutex
- Mutating shared data from threads
- Shared ownership with Arc
- Shared state model
- Concurrency models with threads
- Accessing data from threads
- Customizing threads
- Thread basics
- Concurrency in Rust
- Pitfalls
- User-level
- Kernel-based
- Approaches to concurrency
- Concurrency
- Program execution models
- Concurrency
- Summary
- Serialization and deserialization using serde
- Exploring the std::mem module
- Memory alignment
- Types and memory
- Casting and coercion
- Advanced let destructure
- Match guards
- Advanced match patterns and guards
- Selective privacy
- Re-exports
- Imports
- Modules paths and imports
- Consts in structs enums and traits
- FnOnce closures
- FnMut closures
- Fn closures
- Closures in depth
- Trait rules
- Universal function call syntax
- Trait objects and object safety
- From and Into
- ToOwned
- Borrow and AsRef
- Sized and ?Sized
- Advanced traits
- Cow
- Unions
- Never type ! and diverging functions
- Function types
- Unsized types
- Advanced types
- Implementing a custom iterator
- Iterators
- Dynamic statics using the lazy_static! macro
- Compile time functions – const fn
- Statics
- Constants
- Global values
- When to use &str versus String ?
- Joining strings
- Using strings in functions
- Slicing and dicing strings
- Borrowed strings – &str
- Owned strings – String
- Strings
- Type aliases
- Type inference
- Type clarity and sign distinction in numeric types
- Loop as an expression
- Let statements
- Blocks and expressions
- Type system tidbits
- Advanced Concepts
- Summary
- Custom errors and the Error trait
- User-friendly panics
- Non-recoverable errors
- Early returns and the ? operator
- Converting between Option and Result
- Using combinators
- Common combinators
- Combinators on Option/Result
- Result
- Option
- Recoverable errors
- Error handling prelude
- Error Handling
- Summary
- Uses of interior mutability
- RefCell<T>
- Cell<T>
- Interior mutability
- Rc<T>
- Reference counted smart pointers
- Box<T>
- Types of smart pointers
- Deref and DerefMut
- Drop
- Smart pointers
- Raw pointers
- References – safe pointers
- Pointer types in Rust
- Specifying lifetime bounds on generic types
- Lifetime subtyping
- Multiple lifetimes
- Lifetime in impl blocks
- Lifetimes in user defined types
- Lifetime elision and the rules
- Lifetime parameters
- Lifetimes
- Method types based on borrowing
- Borrowing in action
- Borrowing rules
- Borrowing
- Ownership in action
- Clone
- Copy
- Duplicating types via traits
- Move and copy semantics
- A brief on scopes
- Ownership
- Trifecta of memory safety
- Memory safety
- Memory management pitfalls
- The heap
- The stack
- Approaches to memory allocation
- Memory management and its kinds
- How do programs use memory?
- Programs and memory
- Memory Management and Safety
- Summary
- Trait objects
- Dispatch
- True polymorphism using trait objects
- Exploring standard library traits
- Trait bounds with impl trait syntax
- Using + to compose traits as bounds
- Trait bounds on generic functions and impl blocks
- Trait bounds on types
- Using traits with generics – trait bounds
- Inherited traits
- Associated type traits
- Generic traits
- Simple traits
- Marker traits
- The many forms of traits
- Traits
- Abstracting behavior with traits
- Using generics
- Generic implementations
- Generic types
- Generic functions
- Creating generic types
- Generics
- Type systems and why they matter
- Types Generics and Traits
- Summary
- Continuous integration with Travis CI
- Writing and testing a crate – logic gate simulator
- Benchmarking on stable Rust
- Built-in micro-benchmark harness
- Benchmarks
- Documentation tests
- Doc attributes
- Hosting documentation
- Generating and viewing documentation
- Writing documentation
- Documentation
- Sharing common code
- First integration test
- Integration tests
- Ignoring tests
- Failing tests
- Isolating test code
- Running tests
- First unit test
- Unit tests
- Assertion macros
- Attributes
- Testing primitives
- Organizing tests
- Motivation for testing
- Tests Documentation and Benchmarks
- Summary
- Building a project with Cargo – imgtool
- Setting up a Rust development environment
- Exploring the manifest file – Cargo.toml
- Linting code with clippy
- cargo-outdated
- cargo-deb
- cargo-edit
- cargo-watch
- Subcommands and Cargo installation
- Extending Cargo and tools
- Cargo workspace
- Running examples with Cargo
- Running tests with Cargo
- Cargo and dependencies
- Creating a new Cargo project
- Cargo and crates
- Directory as module
- File as a module
- Nested modules
- Modules
- Package managers
- Managing Projects with Cargo
- Summary
- Exercise – fixing the word counter
- Iterators
- Slices
- Hashmaps
- Vectors
- Tuples
- Arrays
- Collections
- Modules imports and use statements
- Impl blocks for enums
- Impl blocks on structs
- Functions and methods on types
- Enums
- Structs
- User-defined types
- Loops
- Match expressions
- Conditionals and decision making
- Strings
- Closures
- Functions
- Declaring variables and immutability
- Primitive types
- A tour of the language
- Using rustup.rs
- Installing the Rust compiler and toolchain
- What is Rust and why should you care?
- Getting Started with Rust
- Reviews
- Get in touch
- Conventions used
- Download the example code files
- Getting the most out of this book
- What this book covers
- Who this book is for
- Preface
- Packt is searching for authors like you
- About the reviewer
- About the author
- Contributors
- Packt.com
- Why subscribe?
- About Packt
- Mastering Rust Second Edition
- Copyright and Credits
- Title Page
- coverpage
- coverpage
- Title Page
- Copyright and Credits
- Mastering Rust Second Edition
- About Packt
- Why subscribe?
- Packt.com
- Contributors
- About the author
- About the reviewer
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- Getting the most out of this book
- Download the example code files
- Conventions used
- Get in touch
- Reviews
- Getting Started with Rust
- What is Rust and why should you care?
- Installing the Rust compiler and toolchain
- Using rustup.rs
- A tour of the language
- Primitive types
- Declaring variables and immutability
- Functions
- Closures
- Strings
- Conditionals and decision making
- Match expressions
- Loops
- User-defined types
- Structs
- Enums
- Functions and methods on types
- Impl blocks on structs
- Impl blocks for enums
- Modules imports and use statements
- Collections
- Arrays
- Tuples
- Vectors
- Hashmaps
- Slices
- Iterators
- Exercise – fixing the word counter
- Summary
- Managing Projects with Cargo
- Package managers
- Modules
- Nested modules
- File as a module
- Directory as module
- Cargo and crates
- Creating a new Cargo project
- Cargo and dependencies
- Running tests with Cargo
- Running examples with Cargo
- Cargo workspace
- Extending Cargo and tools
- Subcommands and Cargo installation
- cargo-watch
- cargo-edit
- cargo-deb
- cargo-outdated
- Linting code with clippy
- Exploring the manifest file – Cargo.toml
- Setting up a Rust development environment
- Building a project with Cargo – imgtool
- Summary
- Tests Documentation and Benchmarks
- Motivation for testing
- Organizing tests
- Testing primitives
- Attributes
- Assertion macros
- Unit tests
- First unit test
- Running tests
- Isolating test code
- Failing tests
- Ignoring tests
- Integration tests
- First integration test
- Sharing common code
- Documentation
- Writing documentation
- Generating and viewing documentation
- Hosting documentation
- Doc attributes
- Documentation tests
- Benchmarks
- Built-in micro-benchmark harness
- Benchmarking on stable Rust
- Writing and testing a crate – logic gate simulator
- Continuous integration with Travis CI
- Summary
- Types Generics and Traits
- Type systems and why they matter
- Generics
- Creating generic types
- Generic functions
- Generic types
- Generic implementations
- Using generics
- Abstracting behavior with traits
- Traits
- The many forms of traits
- Marker traits
- Simple traits
- Generic traits
- Associated type traits
- Inherited traits
- Using traits with generics – trait bounds
- Trait bounds on types
- Trait bounds on generic functions and impl blocks
- Using + to compose traits as bounds
- Trait bounds with impl trait syntax
- Exploring standard library traits
- True polymorphism using trait objects
- Dispatch
- Trait objects
- Summary
- Memory Management and Safety
- Programs and memory
- How do programs use memory?
- Memory management and its kinds
- Approaches to memory allocation
- The stack
- The heap
- Memory management pitfalls
- Memory safety
- Trifecta of memory safety
- Ownership
- A brief on scopes
- Move and copy semantics
- Duplicating types via traits
- Copy
- Clone
- Ownership in action
- Borrowing
- Borrowing rules
- Borrowing in action
- Method types based on borrowing
- Lifetimes
- Lifetime parameters
- Lifetime elision and the rules
- Lifetimes in user defined types
- Lifetime in impl blocks
- Multiple lifetimes
- Lifetime subtyping
- Specifying lifetime bounds on generic types
- Pointer types in Rust
- References – safe pointers
- Raw pointers
- Smart pointers
- Drop
- Deref and DerefMut
- Types of smart pointers
- Box<T>
- Reference counted smart pointers
- Rc<T>
- Interior mutability
- Cell<T>
- RefCell<T>
- Uses of interior mutability
- Summary
- Error Handling
- Error handling prelude
- Recoverable errors
- Option
- Result
- Combinators on Option/Result
- Common combinators
- Using combinators
- Converting between Option and Result
- Early returns and the ? operator
- Non-recoverable errors
- User-friendly panics
- Custom errors and the Error trait
- Summary
- Advanced Concepts
- Type system tidbits
- Blocks and expressions
- Let statements
- Loop as an expression
- Type clarity and sign distinction in numeric types
- Type inference
- Type aliases
- Strings
- Owned strings – String
- Borrowed strings – &str
- Slicing and dicing strings
- Using strings in functions
- Joining strings
- When to use &str versus String ?
- Global values
- Constants
- Statics
- Compile time functions – const fn
- Dynamic statics using the lazy_static! macro
- Iterators
- Implementing a custom iterator
- Advanced types
- Unsized types
- Function types
- Never type ! and diverging functions
- Unions
- Cow
- Advanced traits
- Sized and ?Sized
- Borrow and AsRef
- ToOwned
- From and Into
- Trait objects and object safety
- Universal function call syntax
- Trait rules
- Closures in depth
- Fn closures
- FnMut closures
- FnOnce closures
- Consts in structs enums and traits
- Modules paths and imports
- Imports
- Re-exports
- Selective privacy
- Advanced match patterns and guards
- Match guards
- Advanced let destructure
- Casting and coercion
- Types and memory
- Memory alignment
- Exploring the std::mem module
- Serialization and deserialization using serde
- Summary
- Concurrency
- Program execution models
- Concurrency
- Approaches to concurrency
- Kernel-based
- User-level
- Pitfalls
- Concurrency in Rust
- Thread basics
- Customizing threads
- Accessing data from threads
- Concurrency models with threads
- Shared state model
- Shared ownership with Arc
- Mutating shared data from threads
- Mutex
- Shared mutability with Arc and Mutex
- RwLock
- Communicating through message passing
- Asynchronous channels
- Synchronous channels
- thread-safety in Rust
- What is thread-safety?
- Traits for thread-safety
- Send
- Sync
- Concurrency using the actor model
- Other crates
- Summary
- Metaprogramming with Macros
- What is metaprogramming?
- When to use and not use Rust macros
- Macros in Rust and their types
- Types of macros
- Creating your first macro with macro_rules!
- Built-in macros in the standard library
- macro_rules! token types
- Repetitions in macros
- A more involved macro – writing a DSL for HashMap initialization
- Macro use case – writing tests
- Exercises
- Procedural macros
- Derive macros
- Debugging macros
- Useful procedural macro crates
- Summary
- Unsafe Rust and Foreign Function Interfaces
- What is safe and unsafe really?
- Unsafe functions and blocks
- Unsafe traits and implementations
- Calling C code from Rust
- Calling Rust code from C
- Using external C/C++ libraries from Rust
- Creating native Python extensions with PyO3
- Creating native extensions in Rust for Node.js
- Summary
- Logging
- What is logging and why do we need it?
- The need for logging frameworks
- Logging frameworks and their key features
- Approaches to logging
- Unstructured logging
- Structured logging
- Logging in Rust
- log – Rust's logging facade
- The env_logger
- log4rs
- Structured logging using slog
- Summary
- Network Programming in Rust
- Network programming prelude
- Synchronous network I/O
- Building a synchronous redis server
- Asynchronous network I/O
- Async abstractions in Rust
- Mio
- Futures
- Tokio
- Building an asynchronous redis server
- Summary
- Building Web Applications with Rust
- Web applications in Rust
- Typed HTTP with Hyper
- Hyper server APIs – building a URL shortener
- hyper as a client – building a URL shortener client
- Web frameworks
- Actix-web basics
- Building a bookmarks API using Actix-web
- Summary
- Interacting with Databases in Rust
- Why do we need data persistence?
- SQLite
- PostgreSQL
- Connection pooling with r2d2
- Postgres and the diesel ORM
- Summary
- Rust on the Web with WebAssembly
- What is WebAssembly?
- Design goals of WebAssembly
- Getting started with WebAssembly
- Trying it out online
- Ways to generate WebAssembly
- Rust and WebAssembly
- Wasm-bindgen
- Other WebAssembly projects
- Rust
- Other languages
- Summary
- Building Desktop Applications with Rust
- Introduction to GUI development
- GTK+ framework
- Building a hacker news app using gtk-rs
- Exercise
- Other emerging GUI frameworks
- Summary
- Debugging
- Introduction to debugging
- Debuggers in general
- Prerequisites for debugging
- Setting up gdb
- A sample program – buggie
- The gdb basics
- Debugger integration with Visual Studio Code
- RR debugger – a quick overview
- Summary
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-07-02 13:36:18