舉報
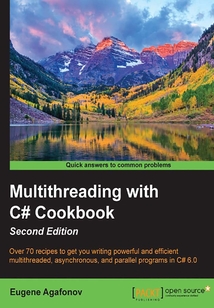
會員
Multithreading with C# Cookbook(Second Edition)
最新章節:
Index
Thisbookisaimedatthosewhoarenewtomultithreadedprogramming,andwhoarelookingforaquickandeasywaytogetstarted.ItisassumedthatyouhavesomeexperienceinC#and.NETalready,andyoushouldalsobefamiliarwithbasiccomputerscienceterminologyandbasicalgorithmsanddatastructures.
目錄(105章)
倒序
- 封面
- 版權信息
- Credits
- About the Author
- About the Reviewers
- www.PacktPub.com
- Preface
- Chapter 1. Threading Basics
- Introduction
- Creating a thread in C#
- Pausing a thread
- Making a thread wait
- Aborting a thread
- Determining a thread state
- Thread priority
- Foreground and background threads
- Passing parameters to a thread
- Locking with a C# lock keyword
- Locking with a Monitor construct
- Handling exceptions
- Chapter 2. Thread Synchronization
- Introduction
- Performing basic atomic operations
- Using the Mutex construct
- Using the SemaphoreSlim construct
- Using the AutoResetEvent construct
- Using the ManualResetEventSlim construct
- Using the CountDownEvent construct
- Using the Barrier construct
- Using the ReaderWriterLockSlim construct
- Using the SpinWait construct
- Chapter 3. Using a Thread Pool
- Introduction
- Invoking a delegate on a thread pool
- Posting an asynchronous operation on a thread pool
- A thread pool and the degree of parallelism
- Implementing a cancellation option
- Using a wait handle and timeout with a thread pool
- Using a timer
- Using the BackgroundWorker component
- Chapter 4. Using the Task Parallel Library
- Introduction
- Creating a task
- Performing basic operations with a task
- Combining tasks
- Converting the APM pattern to tasks
- Converting the EAP pattern to tasks
- Implementing a cancelation option
- Handling exceptions in tasks
- Running tasks in parallel
- Tweaking the execution of tasks with TaskScheduler
- Chapter 5. Using C# 6.0
- Introduction
- Using the await operator to get asynchronous task results
- Using the await operator in a lambda expression
- Using the await operator with consequent asynchronous tasks
- Using the await operator for the execution of parallel asynchronous tasks
- Handling exceptions in asynchronous operations
- Avoiding the use of the captured synchronization context
- Working around the async void method
- Designing a custom awaitable type
- Using the dynamic type with await
- Chapter 6. Using Concurrent Collections
- Introduction
- Using ConcurrentDictionary
- Implementing asynchronous processing using ConcurrentQueue
- Changing asynchronous processing order with ConcurrentStack
- Creating a scalable crawler with ConcurrentBag
- Generalizing asynchronous processing with BlockingCollection
- Chapter 7. Using PLINQ
- Introduction
- Using the Parallel class
- Parallelizing a LINQ query
- Tweaking the parameters of a PLINQ query
- Handling exceptions in a PLINQ query
- Managing data partitioning in a PLINQ query
- Creating a custom aggregator for a PLINQ query
- Chapter 8. Reactive Extensions
- Introduction
- Converting a collection to an asynchronous Observable
- Writing custom Observable
- Using the Subject type family
- Creating an Observable object
- Using LINQ queries against an observable collection
- Creating asynchronous operations with Rx
- Chapter 9. Using Asynchronous I/O
- Introduction
- Working with files asynchronously
- Writing an asynchronous HTTP server and client
- Working with a database asynchronously
- Calling a WCF service asynchronously
- Chapter 10. Parallel Programming Patterns
- Introduction
- Implementing Lazy-evaluated shared states
- Implementing Parallel Pipeline with BlockingCollection
- Implementing Parallel Pipeline with TPL DataFlow
- Implementing Map/Reduce with PLINQ
- Chapter 11. There's More
- Introduction
- Using a timer in a Universal Windows Platform application
- Using WinRT from usual applications
- Using BackgroundTask in Universal Windows Platform applications
- Running a .NET Core application on OS X
- Running a .NET Core application on Ubuntu Linux
- Index 更新時間:2021-07-09 19:35:50
推薦閱讀
- Visual Studio 2012 Cookbook
- HBase從入門到實戰
- RTC程序設計:實時音視頻權威指南
- x86匯編語言:從實模式到保護模式(第2版)
- 程序員考試案例梳理、真題透解與強化訓練
- Web Application Development with MEAN
- 你不知道的JavaScript(中卷)
- Oracle Exadata專家手冊
- 大學計算機基礎實驗指導
- Learning Node.js for .NET Developers
- 小程序從0到1:微信全棧工程師一本通
- Qt 4開發實踐
- Java程序設計實用教程(第2版)
- Node.js實戰:分布式系統中的后端服務開發
- 零基礎學SQL(升級版)
- HTML5 Canvas核心技術:圖形、動畫與游戲開發
- 美麗洞察力:從化妝品行業看顧客需求洞察
- 精通Oracle 12c 數據庫管理
- 狼書(卷2):Node.js Web應用開發
- TensorFlow程序設計
- HTML5與CSS3權威指南(第2版·下冊)
- Bootstrap for ASP.NET MVC(Second Edition)
- Balsamiq Wireframes Quickstart Guide
- 數據結構:Python語言描述
- PLC編程入門及工程實例
- Heroku Cookbook
- Microsoft SharePoint 2013 Disaster Recovery Guide
- 同構:編程中的數學
- Haskell High Performance Programming
- Visual Basic 2010入門經典