舉報
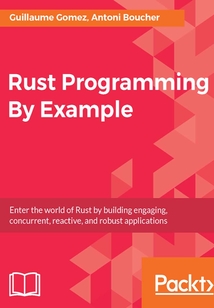
會員
Rust Programming By Example
ThisbookisforsoftwaredevelopersinterestedinsystemlevelandapplicationprogrammingwhoarelookingforaquickentryintousingRustandunderstandingthecorefeaturesoftheRustProgramming.It’sassumedthatyouhaveabasicunderstandingofJava,C#,Ruby,Python,orJavaScript.
目錄(311章)
倒序
- 封面
- 版權信息
- Packt Upsell
- Why subscribe?
- PacktPub.com
- Contributors
- About the authors
- About the reviewers
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Reviews
- Basics of Rust
- Getting to know Rust
- Installing Rust
- Windows
- Linux/Mac
- Test your installation
- Documentation and reference
- Main function
- Variables
- Built-in data types
- Integer types
- Floating-point types
- Boolean type
- Character type
- Control flow
- Writing a condition
- Creating while loops
- Creating functions
- Creating structures
- References
- Clone types
- Copy types
- Mutable references
- Methods
- Constructors
- Tuples
- Enumerations
- Pattern matching
- Irrefutable patterns
- Traits
- Default methods
- Associated types
- Rules
- Generics
- The Option type
- Arrays
- Slices
- For loops
- Macros
- Multiple pattern rules
- Repetitions
- Optional quantifier
- Summary
- Starting with SDL
- Understanding Rust crates
- Installing SDL2
- Installing SDL2 on Linux
- Installing SDL2 on Mac
- Installing SDL2 on Windows
- Windows with Build Script
- Windows (MinGW)
- Windows (MSVC)
- Setting up your Rust project
- Cargo and crates.io
- The docs.rs documentation
- Back to our Cargo.toml file
- Rust's modules
- Tetris
- Creating a window
- Drawing
- Playing with Options
- Solution
- Loading images
- Installing SDL2_image on Mac
- Installing SDL2_image on Linux
- Installing SDL2_image on Windows
- Playing with features
- Playing with images
- Handling files
- Saving/loading high scores
- Iterators
- Reading formatted data from files
- Summary
- Events and Basic Game Mechanisms
- Writing Tetris
- Tetrimino
- Creating tetriminos
- Generating a tetrimino
- Rotating a tetrimino
- Tetris struct
- Interacting with the game map
- SDL events
- Score level lines sent
- Levels and lines sent
- Highscores loading/overwriting
- Summary
- Adding All Game Mechanisms
- Getting started with game mechanisms
- Rendering UI
- Rendering initialization
- Rendering
- Playing with fonts
- Install on OS X
- Install on Linux
- Other system/package manager
- Loading font
- Summary
- Creating a Music Player
- Installing the prerequisite
- Installing GTK+ on Linux
- Installing GTK+ on Mac
- Installing GTK+ on Windows
- Creating your first window
- Closure
- Preventing the default behavior of an event
- Creating a toolbar
- Stock item
- Improving the organization of the application
- Adding tool button events
- Lifetime
- Ownership
- Containers
- Types of containers
- The Box container
- Adding a playlist
- The MVC pattern
- Opening MP3 files
- Reference-counting pointer
- ID3— MP3 metadata
- Opening files with a file dialog
- Deleting a song
- Displaying the cover when playing a song
- Summary
- Implementing the Engine of the Music Player
- Installing the dependencies
- Installing dependencies on Linux
- Installing dependencies on Mac
- Installing dependencies on Windows
- Decoding MP3 files
- Adding dependencies
- Implementing an MP3 decoder
- Getting the frame samples
- Playing music
- Event loop
- Atomic reference counting
- Mutual exclusion
- Send trait
- Sync trait
- Lock-free data structures
- Playing music
- Mutex guard
- RAII
- Using the music player
- Pausing and resuming the song
- Interior mutability
- Showing the progression of the song
- Improving CPU usage
- Condition variable
- Showing the song's current time
- Loading and saving the playlist
- Saving a playlist
- Loading a playlist
- Using gstreamer for playback
- Summary
- Music Player in a More Rusty Way with Relm
- Reasons to use relm instead of gtk-rs directly
- State mutation
- Asynchronous user interface
- Creating custom widgets
- Creating a window with relm
- Installing Rust nightly
- Widget
- Model
- Messages
- View
- Properties
- Events
- Code generation
- Update function
- Adding child widgets
- One-way data binding
- Post-initialization of the view
- Dialogs
- Other methods
- Playlist
- Model parameter
- Adding a relm widget
- Communicating between widgets
- Communicating with the same widget
- Emit
- With different widgets
- Handle messages from a relm widget
- Syntax sugar to send a message to another relm widget
- Playing music
- Computing the song duration
- Using relm on stable Rust
- Relm widgets data binding
- Summary
- Understanding FTP
- File transfer protocol
- Introduction to FTP
- Implementing simple chunks of commands
- Starting with basics
- Commands implementation
- Implementing the SYST command
- Implementing the USER command
- Implementing the NOOP command
- Implementing the PWD command
- Implementing the TYPE command
- Implementing the LIST command
- Implementing the PASV command
- Back to the LIST command
- Implementing the CWD command
- Implementing the CDUP command
- Full implementation of the LIST command
- Implementing the MKD command
- Implementing the RMD command
- Testing it
- Summary
- Implementing an Asynchronous FTP Server
- Advantages of asynchronous IO
- Disadvantages of asynchronous IO
- Creating the new project
- Using Tokio
- Tokio event loop
- Using futures
- Handling errors
- Unwrapping
- Custom error type
- Displaying the error
- Composing error types
- The ? operator revisited
- Starting the Tokio event loop
- Starting the server
- Handling clients
- Handling commands
- FTP codec
- Decoding FTP commands
- Encoding FTP commands
- Handling commands
- Managing the current working directory
- Printing the current directory
- Changing the current directory
- Setting the transfer type
- Entering passive mode
- Bytes codec
- Decoding data bytes
- Encoding data bytes
- Quitting
- Creating directories
- Removing directories
- Summary
- Implementing Asynchronous File Transfer
- Listing files
- Downloading a file
- Uploading files
- Going further!
- Configuration
- Securing the config.toml access
- Unit tests
- Backtraces
- Testing failures
- Ignoring tests
- Integration tests
- Teardown
- Print output to stdout
- Documentation
- Documenting a crate
- Documenting a module
- Headers
- Code blocks
- Documenting an enumeration (or any type with public fields)
- Generating the documentation
- Warning about public items without documentation
- Hiding items from the documentation
- Documentation tests
- Tags
- ignore
- compile_fail
- no_run
- should_panic
- Combining flags?
- About the doc blocks themselves
- Hiding code blocks lines
- Fuzzing tests
- Summary
- Rust Best Practices
- Rust best practices
- Slices
- API tips and improvements
- Explaining the Some function
- Using the Path function
- Usage tips
- Builder pattern
- Playing with mutable borrows
- Playing with moves
- Code readability
- Big number formatting
- Specifying types
- Matching
- Summary
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-07-02 19:13:51
推薦閱讀
- 普通高校中文學科基礎教材古典文獻學基礎
- 全民閱讀組織活動讀本
- 國內圖書情報知識圖譜實證研究
- Learning Robotics using Python
- 檔案檢索: 理論與方法
- 檔案利用與服務
- 構筑閱讀天堂:圖書館服務設計探索
- 國際集郵聯合會(FIP)集郵展覽評審規則
- 移動社交時代的電子閱讀
- 文書與檔案管理實務
- 中國人民大學復印報刊資料轉載指數排名研究報告2018(中國人民大學研究報告系列)
- 天南學術(第一輯)
- 武大老照片
- 李一氓文存(第三卷):題跋·詩聯·劇本
- 浩蕩游絲:何焯與清代的批校文化(精裝)
- 中國人民大學復印報刊資料轉載指數排名研究報告(2019)
- 看法與說法(全四冊)
- 北京大學中國古文獻研究中心集刊·第十六輯
- 西南少數民族經濟古籍文獻研究
- 人文通識講演錄:文化卷
- 社會經濟
- 元代文獻與文化研究(第一輯)
- 如何查找文獻(第二版)
- 中華武術
- 現代圖書館學熱點研究
- 圖書館創新與現代管理研究
- 珞珈蘭臺文集
- 國家圖書館業務管理機制研究
- 數字時代的圖書
- 全民健身組織活動讀本