首頁 > 計算機(jī)網(wǎng)絡(luò) >
數(shù)據(jù)庫
> Hands-On Data Structures and Algorithms with Rust最新章節(jié)目錄
舉報
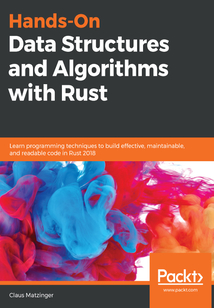
會員
Hands-On Data Structures and Algorithms with Rust
Rusthascomealongwayandisnowutilizedinseveralcontexts.Itskeystrengthsareitssoftwareinfrastructureandresource-constrainedapplications,includingdesktopapplications,servers,andperformance-criticalapplications,notforgettingitsimportanceinsystems'programming.ThisbookwillbeyourguideasittakesyouthroughimplementingclassicdatastructuresandalgorithmsinRust,helpingyoutogetupandrunningasaconfidentRustprogrammer.ThebookbeginswithanintroductiontoRustdatastructuresandalgorithms,whilealsocoveringessentiallanguageconstructs.Youwilllearnhowtostoredatausinglinkedlists,arrays,stacks,andqueues.Youwillalsolearnhowtoimplementsortingandsearchingalgorithms.Youwilllearnhowtoattainhighperformancebyimplementingalgorithmstostringdatatypesandimplementhashstructuresinalgorithmdesign.Thebookwillexaminealgorithmanalysis,includingBruteForcealgorithms,Greedyalgorithms,DivideandConqueralgorithms,DynamicProgramming,andBacktracking.Bytheendofthebook,youwillhavelearnedhowtobuildcomponentsthatareeasytounderstand,debug,anduseindifferentapplications.
目錄(301章)
倒序
- coverpage
- Title Page
- Copyright and Credits
- Hands-On Data Structures and Algorithms with Rust
- About Packt
- Why subscribe?
- Packt.com
- Foreword
- Contributors
- About the author
- About the reviewer
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the color images
- Download the example code files
- Conventions used
- Get in touch
- Reviews
- Hello Rust!
- Rust in 2018
- The 2018 edition
- The Rust language
- Objects and behavior
- Going wrong
- Macros
- Unsafe
- Borrowing and ownership
- Exceptional lifetimes
- Multiple owners
- Concurrency and mutability
- Immutable variables
- Shadowing
- Interior mutability
- Moving data
- Sharing data
- Send and Sync
- Deeper into Rust
- Requests for Comments (RFCs)
- Summary
- Questions
- Further reading
- Cargo and Crates
- Cargo
- Project configuration
- The manifest – Cargo.toml
- Package
- Profiles
- Dependencies
- Dependencies – Cargo.lock
- Commands
- The compile and run commands
- Testing
- Third-party subcommands
- Crates
- Rust libraries and binaries
- Static and dynamic libraries
- Linking and interoperability
- FFI
- Wasm
- The main repository – crates.io
- Publishing
- Summary
- Questions
- Further reading
- Storing Efficiently
- Heaps and stacks
- Sized and unsized
- Generics
- Accessing the box
- Copying and cloning
- Immutable storage
- States and reasoning
- Concurrency and performance
- Summary
- Questions
- Further reading
- Lists Lists and More Lists
- Linked lists
- A transaction log
- Adding entries
- Log replay
- After use
- Wrap up
- Upsides
- Downsides
- Doubly linked list
- A better transaction log
- Examining the log
- Reverse
- Wrap up
- Upsides
- Downsides
- Skip lists
- The best transaction log
- The list
- Adding data
- Leveling up
- Jumping around
- Thoughts and discussion
- Upsides
- Downsides
- Dynamic arrays
- Favorite transactions
- Internal arrays
- Quick access
- Wrap up
- Upsides
- Downsides
- Summary
- Questions
- Further reading
- Robust Trees
- Binary search tree
- IoT device management
- More devices
- Finding the right one
- Finding all devices
- Wrap up
- Upsides
- Downsides
- Red-black tree
- Better IoT device management
- Even more devices
- Balancing the tree
- Finding the right one now
- Wrap up
- Upsides
- Downsides
- Heaps
- A huge inbox
- Getting messages in
- Taking messages out
- Wrap up
- Upsides
- Downsides
- Trie
- More realistic IoT device management
- Adding paths
- Walking
- Wrap up
- Upsides
- Downsides
- B-Tree
- An IoT database
- Adding stuff
- Searching for stuff
- Walking the tree
- Wrap up
- Upsides
- Downsides
- Graphs
- The literal Internet of Things
- Neighborhood search
- The shortest path
- Wrap up
- Upsides
- Downsides
- Summary
- Questions
- Exploring Maps and Sets
- Hashing
- Create your own
- Message digestion
- Wrap up
- Maps
- A location cache
- The hash function
- Adding locations
- Fetching locations
- Wrap up
- Upsides
- Downsides
- Sets
- Storing network addresses
- Networked operations
- Union
- Intersection
- Difference
- Wrap up
- Upsides
- Downsides
- Summary
- Questions
- Further reading
- Collections in Rust
- Sequences
- Vec<T> and VecDeque<T>
- Architecture
- Insert
- Look up
- Remove
- LinkedList<T>
- Architecture
- Insert
- Look up
- Remove
- Wrap up
- Maps and sets
- HashMap and HashSet
- Architecture
- Insert
- Lookup
- Remove
- BTreeMap and BTreeSet
- Architecture
- Insert
- Look up
- Remove
- Wrap up
- Summary
- Questions
- Further reading
- Algorithm Evaluation
- The Big O notation
- Other people's code
- The Big O
- Asymptotic runtime complexity
- Making your own
- Loops
- Recursion
- Complexity classes
- O(1)
- O(log(n))
- O(n)
- O(n log(n))
- O(n2)
- O(2n)
- Comparison
- In the wild
- Data structures
- Everyday things
- Exotic things
- Summary
- Questions
- Further reading
- Ordering Things
- From chaos to order
- Bubble sort
- Shell sort
- Heap sort
- Merge sort
- Quicksort
- Summary
- Questions
- Further reading
- Finding Stuff
- Finding the best
- Linear searches
- Jump search
- Binary searching
- Wrap up
- Summary
- Questions
- Further reading
- Random and Combinatorial
- Pseudo-random numbers
- LCG
- Wichmann-Hill
- The rand crate
- Back to front
- Packing bags or the 0-1 knapsack problem
- N queens
- Advanced problem solving
- Dynamic programming
- The knapsack problem improved
- Metaheuristic approaches
- Example metaheuristic – genetic algorithms
- Summary
- Questions
- Further reading
- Algorithms of the Standard Library
- Slicing and iteration
- Iterator
- Slices
- Search
- Linear search
- Binary search
- Sorting
- Stable sorting
- Unstable sorting
- Summary
- Questions
- Further reading
- Assessments
- Chapter 1
- Chapter 2
- Chapter 3
- Chapter 4
- Chapter 5
- Chapter 6
- Chapter 7
- Chapter 8
- Chapter 9
- Chapter 10
- Chapter 11
- Chapter 12
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-07-02 14:12:31
推薦閱讀
- GitHub Essentials
- Java Data Science Cookbook
- 虛擬化與云計算
- Effective Amazon Machine Learning
- 區(qū)塊鏈通俗讀本
- Enterprise Integration with WSO2 ESB
- WS-BPEL 2.0 Beginner's Guide
- 數(shù)據(jù)庫程序員面試筆試真題庫
- 數(shù)據(jù)中心數(shù)字孿生應(yīng)用實踐
- 高維數(shù)據(jù)分析預(yù)處理技術(shù)
- Solaris操作系統(tǒng)原理實驗教程
- 中文版Access 2007實例與操作
- Access數(shù)據(jù)庫開發(fā)從入門到精通
- Deep Learning with R for Beginners
- 大數(shù)據(jù)隱私保護(hù)技術(shù)與治理機(jī)制研究
- Artificial Intelligence for Big Data
- 成功之路:ORACLE 11g學(xué)習(xí)筆記
- Unity 4.x Game AI Programming
- 敏捷數(shù)據(jù)分析工具箱:深入解析ADW+OAC
- Hands-On Big Data Analytics with PySpark
- 邊緣計算使能工業(yè)互聯(lián)網(wǎng)
- 計算機(jī)視覺之深度學(xué)習(xí):使用TensorFlow和Keras訓(xùn)練高級神經(jīng)網(wǎng)絡(luò)
- CDA數(shù)據(jù)分析實務(wù)
- MySQL數(shù)據(jù)庫基礎(chǔ)實例教程
- MySQL 8 Cookbook(中文版)
- 智能數(shù)據(jù)治理:基于大模型、知識圖譜
- 以太坊技術(shù)詳解與實戰(zhàn)
- 檢索匹配:深度學(xué)習(xí)在搜索、廣告、推薦系統(tǒng)中的應(yīng)用
- 數(shù)據(jù)庫技術(shù)及應(yīng)用(Access)實驗指導(dǎo)與習(xí)題集
- Hudson 3 Essentials