舉報
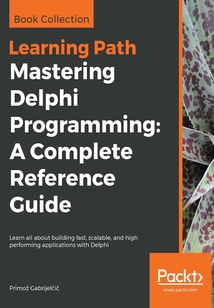
會員
Mastering Delphi Programming:A Complete Reference Guide
Delphiisacross-platformIntegratedDevelopmentEnvironment(IDE)thatsupportsrapidapplicationdevelopmentformostoperatingsystems,includingMicrosoftWindows,iOS,andnowLinuxwithRADStudio10.2.IfyouknowhowtousethefeaturesofDelphi,youcaneasilycreatescalableapplicationsinnotime.ThisLearningPathbeginsbyexplaininghowtofindperformancebottlenecksandapplythecorrectalgorithmtofixthem.You'llbrushupontricks,techniques,andbestpracticestosolvecommondesignandarchitecturalchallenges.Then,you'llseehowtoleverageexternallibrariestowritebetter-performingprograms.You'llalsolearnabouttheeightmostimportantpatternsthat'llenableyoutodevelopandimprovetheinterfacebetweenitemsandharmonizesharedmemorieswithinthreads.Asyouprogress,you'llalsodelveintoimprovingtheperformanceofyourcodeandmasteringcross-platformRTLimprovements.BytheendofthisLearningPath,you'llbeabletoaddresscommondesignproblemsandfeelconfidentwhilebuildingscalableprojects.ThisLearningPathincludescontentfromthefollowingPacktproducts:DelphiHighPerformancebyPrimo?Gabrijel?i?.Hands-OnDesignPatternswithDelphibyPrimo?Gabrijel?i?.
目錄(256章)
倒序
- coverpage
- Title Page
- Copyright and Credits
- Mastering Delphi Programming: A Complete Reference Guide
- About Packt
- Why subscribe?
- PacktPub.com
- Contributors
- About the author
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- Download the example code files
- Conventions used
- Get in touch
- Reviews
- About Performance
- What is performance?
- Different types of speed
- Algorithm complexity
- Big O and Delphi data structures
- Data structures in practice
- Mr. Smith's first program
- Looking at code through the Big O eyes
- Don't guess measure!
- Profiling with TStopwatch
- Profilers
- AsmProfiler
- Sampling Profiler
- AQTime
- Nexus Quality Suite
- Summary
- Fixing the Algorithm
- Responsive user interfaces
- Updating a progress bar
- Bulk updates
- Virtual display
- Caching
- Dynamic cache
- Speeding up SlowCode
- Summary
- Fine-Tuning the Code
- Delphi compiler settings
- Code inlining control
- Optimization
- Record field alignment
- Assertions
- Overflow checking
- Range checking
- Extracting common expressions
- The helpful CPU window
- Behind the scenes
- A plethora of types
- Simple types
- Strings
- Arrays
- Records
- Classes
- Interfaces
- Optimizing method calls
- Parameter passing
- Method inlining
- The magic of pointers
- Going the assembler way
- Returning to SlowCode
- Summary
- Memory Management
- Optimizing strings and array allocations
- Memory management functions
- Dynamic record allocation
- FastMM internals
- Memory allocation in a parallel world
- Replacing the default memory manager
- ScaleMM
- TBBMalloc
- Fine-tuning SlowCode
- Summary
- Getting Started with the Parallel World
- Processes and threads
- When to parallelize the code?
- Most common problems
- Never access UI from a background thread
- Simultaneous reading and writing
- Sharing a variable
- Synchronization
- Critical sections
- Other locking mechanisms
- A short note on coding style
- Shared data with built-in locking
- Interlocked operations
- Object life cycle
- Communication
- Windows messages
- Synchronize and Queue
- Polling
- Performance
- Third-party libraries
- Summary
- Working with Parallel Tools
- TThread
- Advanced TThread
- Setting up a communication channel
- Sending messages from a thread
- Implementing a timer
- Summary
- Exploring Parallel Practices
- Tasks and patterns
- Variable capturing
- Tasks
- Exceptions in tasks
- Parallelizing a loop
- Thread pooling
- Async/Await
- Join
- Join/Await
- Future
- Parallel for
- Pipelines
- Creating the pipeline
- Stages
- Displaying the result and shutting down
- Summary
- Using External Libraries
- Using object files
- Object file formats
- Object file linking in practice
- Using C++ libraries
- Using a proxy DLL in Delphi
- Summary
- Introduction to Patterns
- Patterns in programming
- Patterns are useful
- Delphi idioms – Creating and destroying an object
- Gang of Four started it all
- Don't inherit – compose!
- Pattern taxonomy
- Creational patterns
- Structural patterns
- Behavioral patterns
- Concurrency patterns
- Criticism
- Anti-patterns
- Design principles
- SOLID
- Don't repeat yourself
- KISS and YAGNI
- Summary
- Singleton Dependency Injection Lazy Initialization and Object Pool
- Singleton
- NewInstance
- Lateral thinking
- Dependency injection
- From classes to interfaces
- Using a factory method
- Wrapping up
- Lazy initialization
- Using Spring
- Object pool
- Stock quote connection pool
- Summary
- Factory Method Abstract Factory Prototype and Builder
- Factory method
- Painter
- Modernizing the factory method pattern
- Abstract factory
- Prototype
- Cloning records
- Cloning objects
- Delphi idioms – Assign and AssignTo
- Serialization
- Builder
- Idioms – Fluent interfaces
- Summary
- Composite Flyweight Marker Interface and Bridge
- Composite
- Child management
- Flyweight
- String interning
- A practical example
- Delphi idioms – comparers and hashers
- Marker interface
- Delphi idioms – attributes
- Markers and attributes
- Bridge
- Bridged painting
- Summary
- Adapter Proxy Decorator and Facade
- Selecting an appropriate structural pattern
- Adapter
- Wrapping a class
- Wrapping an interface
- Implementing a queue with a list
- Proxy
- Delphi idioms – replacing components in runtime
- Smart pointers
- Unit testing with mock objects
- Decorator
- Decorating streams
- Delphi idioms – helpers
- Facade
- Summary
- Nullable Value Template Method Command and State
- Null object
- Template method
- Calculating the average value
- Inversion of control
- Command
- Command-based editor
- Creating commands
- Commands
- Invoker
- Client
- Macros
- Cloning
- State
- Unquoting a string
- Summary
- Iterator Visitor Observer and Memento
- Iterator
- Delphi idioms – iterating with for..in
- Implementing custom enumerators
- Using an iterator interface
- Visitor
- Observer
- Observing with Spring
- Memento
- Summary
- Locking Patterns
- Delphi idioms – parallel programming
- Lock
- Custom locking mechanism
- Lock striping
- Single bit locks
- Delphi idioms – bitwise operators
- Double-checked locking
- Optimistic locking
- Readers-writer lock
- Comparing reader-writer implementations
- Summary
- Thread pool Messaging Future and Pipeline
- Thread pool
- Idiosyncrasies of Delphi's TThreadPool
- Messaging
- Windows messages
- Queue and Synchronize
- Polling
- Future
- Pipeline
- Web spider
- Filter stage
- Downloader stage
- Parser stage
- Summary
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-06-24 12:34:13
推薦閱讀
- FPGA從入門到精通(實戰篇)
- 龍芯應用開發標準教程
- Creating Dynamic UI with Android Fragments
- BeagleBone By Example
- Intel FPGA/CPLD設計(高級篇)
- Unity 5.x Game Development Blueprints
- Manage Partitions with GParted How-to
- 微軟互聯網信息服務(IIS)最佳實踐 (微軟技術開發者叢書)
- 筆記本電腦使用、維護與故障排除從入門到精通(第5版)
- Blender Quick Start Guide
- 數字媒體專業英語(第2版)
- Blender Game Engine:Beginner's Guide
- FPGA實驗實訓教程
- Mastering Quantum Computing with IBM QX
- 基于S5PV210處理器的嵌入式開發完全攻略
- 現代多媒體技術及應用
- 計算機組裝與維護
- Exceptional C++:47個C++工程難題、編程問題和解決方案(中文版)
- Arduino項目開發:智能控制
- Sketchbook Pro Digital Painting Essentials
- 51單片機典型模塊開發查詢手冊
- Nginx應用與運維實戰
- 物聯網智能終端設計及工程實例
- 超炫的30個單片機顯示驅動項目
- 計算機技能大賽指導:調試維修
- TensorFlow 1.x Deep Learning Cookbook
- 打印機維修寶典
- FPGA軟件測試與評價技術
- CXL體系結構:高速互連的原理解析與實踐
- Salesforce CRM:The Definitive Admin Handbook(Second Edition)