舉報
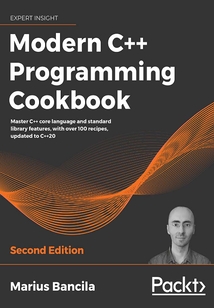
會員
Modern C++ Programming Cookbook
最新章節:
Index
C++hascomealongwaytobeoneofthemostwidelyusedgeneral-purposelanguagesthatisfast,efficient,andhigh-performanceatitscore.TheupdatedsecondeditionofModernC++ProgrammingCookbookaddressesthelatestfeaturesofC++20,suchasmodules,concepts,coroutines,andthemanyadditionstothestandardlibrary,includingrangesandtextformatting.Thebookisorganizedintheformofpracticalrecipescoveringawiderangeofproblemsfacedbymoderndevelopers.ThebookalsodelvesintothedetailsofallthecoreconceptsinmodernC++programming,suchasfunctionsandclasses,iteratorsandalgorithms,streamsandthefilesystem,threadingandconcurrency,smartpointersandmovesemantics,andmanyothers.Itgoesintotheperformanceaspectsofprogrammingindepth,teachingdevelopershowtowritefastandleancodewiththehelpofbestpractices.Furthermore,thebookexploresusefulpatternsanddelvesintotheimplementationofmanyidioms,includingpimpl,namedparameter,andattorney-client,teachingtechniquessuchasavoidingrepetitionwiththefactorypattern.Thereisalsoachapterdedicatedtounittesting,whereyouareintroducedtothreeofthemostwidelyusedlibrariesforC++:Boost.Test,GoogleTest,andCatch2.Bytheendofthebook,youwillbeabletoeffectivelyleveragethefeaturesandtechniquesofC++11/14/17/20programmingtoenhancetheperformance,scalability,andefficiencyofyourapplications.
目錄(160章)
倒序
- 封面
- 版權信息
- Why subscribe?
- Contributors About the author
- About the reviewer
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Get in touch
- 1 Learning Modern Core Language Features
- Using auto whenever possible
- Creating type aliases and alias templates
- Understanding uniform initialization
- Understanding the various forms of non-static member initialization
- Controlling and querying object alignment
- Using scoped enumerations
- Using override and final for virtual methods
- Using range-based for loops to iterate on a range
- Enabling range-based for loops for custom types
- Using explicit constructors and conversion operators to avoid implicit conversion
- Using unnamed namespaces instead of static globals
- Using inline namespaces for symbol versioning
- Using structured bindings to handle multi-return values
- Simplifying code with class template argument deduction
- 2 Working with Numbers and Strings
- Converting between numeric and string types
- Limits and other properties of numeric types
- Generating pseudo-random numbers
- Initializing all bits of internal state of a pseudo-random number generator
- Creating cooked user-defined literals
- Creating raw user-defined literals
- Using raw string literals to avoid escaping characters
- Creating a library of string helpers
- Verifying the format of a string using regular expressions
- Parsing the content of a string using regular expressions
- Replacing the content of a string using regular expressions
- Using string_view instead of constant string references
- Formatting text with std::format
- Using std::format with user-defined types
- 3 Exploring Functions
- Defaulted and deleted functions
- Using lambdas with standard algorithms
- Using generic and template lambdas
- Writing a recursive lambda
- Writing a function template with a variable number of arguments
- Using fold expressions to simplify variadic function templates
- Implementing the higher-order functions map and fold
- Composing functions into a higher-order function
- Uniformly invoking anything callable
- 4 Preprocessing and Compilation
- Conditionally compiling your source code
- Using the indirection pattern for preprocessor stringification and concatenation
- Performing compile-time assertion checks with static_assert
- Conditionally compiling classes and functions with enable_if
- Selecting branches at compile time with constexpr if
- Providing metadata to the compiler with attributes
- 5 Standard Library Containers Algorithms and Iterators
- Using vector as a default container
- Using bitset for fixed-size sequences of bits
- Using vector<bool> for variable-size sequences of bits
- Using the bit manipulation utilities
- Finding elements in a range
- Sorting a range
- Initializing a range
- Using set operations on a range
- Using iterators to insert new elements into a container
- Writing your own random-access iterator
- Container access with non-member functions
- 6 General-Purpose Utilities
- Expressing time intervals with chrono::duration
- Working with calendars
- Converting times between time zones
- Measuring function execution time with a standard clock
- Generating hash values for custom types
- Using std::any to store any value
- Using std::optional to store optional values
- Using std::variant as a type-safe union
- Visiting an std::variant
- Using std::span for contiguous sequences of objects
- Registering a function to be called when a program exits normally
- Using type traits to query properties of types
- Writing your own type traits
- Using std::conditional to choose between types
- 7 Working with Files and Streams
- Reading and writing raw data from/to binary files
- Reading and writing objects from/to binary files
- Using localized settings for streams
- Using I/O manipulators to control the output of a stream
- Using monetary I/O manipulators
- Using time I/O manipulators
- Working with filesystem paths
- Creating copying and deleting files and directories
- Removing content from a file
- Checking the properties of an existing file or directory
- Enumerating the content of a directory
- Finding a file
- 8 Leveraging Threading and Concurrency
- Working with threads
- Synchronizing access to shared data with mutexes and locks
- Avoiding using recursive mutexes
- Handling exceptions from thread functions
- Sending notifications between threads
- Using promises and futures to return values from threads
- Executing functions asynchronously
- Using atomic types
- Implementing parallel map and fold with threads
- Implementing parallel map and fold with tasks
- Implementing parallel map and fold with standard parallel algorithms
- Using joinable threads and cancellation mechanisms
- Using thread synchronization mechanisms
- 9 Robustness and Performance
- Using exceptions for error handling
- Using noexcept for functions that do not throw exceptions
- Ensuring constant correctness for a program
- Creating compile-time constant expressions
- Creating immediate functions
- Performing correct type casts
- Using unique_ptr to uniquely own a memory resource
- Using shared_ptr to share a memory resource
- Implementing move semantics
- Consistent comparison with the operator <=>
- 10 Implementing Patterns and Idioms
- Avoiding repetitive if...else statements in factory patterns
- Implementing the pimpl idiom
- Implementing the named parameter idiom
- Separating interfaces and implementations with the non-virtual interface idiom
- Handling friendship with the attorney-client idiom
- Static polymorphism with the curiously recurring template pattern
- Implementing a thread-safe singleton
- 11 Exploring Testing Frameworks
- Getting started with Boost.Test
- Writing and invoking tests with Boost.Test
- Asserting with Boost.Test
- Using fixtures in Boost.Test
- Controlling outputs with Boost.Test
- Getting started with Google Test
- Writing and invoking tests with Google Test
- Asserting with Google Test
- Using test fixtures with Google Test
- Controlling output with Google Test
- Getting started with Catch2
- Writing and invoking tests with Catch2
- Asserting with Catch2
- Controlling output with Catch2
- 12 C++20 Core Features
- Working with modules
- Understanding module partitions
- Specifying requirements on template arguments with concepts
- Using requires expressions and clauses
- Iterating over collections with the ranges library
- Creating your own range view
- Creating a coroutine task type for asynchronous computations
- Creating a coroutine generator type for sequences of values
- Bibliography
- Websites
- Articles and books
- Other Books You May Enjoy
- Leave a review - let other readers know what you think
- Index 更新時間:2021-06-11 18:22:41
推薦閱讀
- Mastering OpenLayers 3
- The Complete Rust Programming Reference Guide
- Cassandra Design Patterns(Second Edition)
- C語言程序設計教程
- C程序設計實踐教程
- 蘋果的產品設計之道:創建優秀產品、服務和用戶體驗的七個原則
- Mastering Concurrency in Python
- 零基礎C#學習筆記
- SSH框架企業級應用實戰
- Instant GLEW
- Python Social Media Analytics
- Professional JavaScript
- Elastix Unified Communications Server Cookbook
- Android熱門應用開發詳解
- C語言程序設計實驗指導教程
- C語言從入門到精通(微視頻精編版)
- Visual Basic程序設計
- ServiceStack 4 Cookbook
- Instant Ubuntu
- Learning PowerShell DSC
- ROS機器人程序設計
- 軟技能:代碼之外的生存指南
- Python數據科學指南
- Clojure Web開發實戰
- INSTANT Spring Tool Suite
- Maven Essentials
- Go語言高級開發與實戰
- Python網絡爬蟲技術
- Raspberry Pi Android Projects
- Testing with F#