舉報
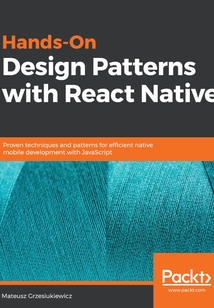
會員
Hands-On Design Patterns with React Native
ReactNativehelpsdevelopersreusecodeacrossdifferentmobileplatformslikeiOSandAndroid.ThisbookwillshowyoueffectivedesignpatternsintheReactNativeworldandwillmakeyoureadyforprofessionaldevelopmentinbigteams.ThebookwillfocusonlyonthepatternsthatarerelevanttoJavaScript,ECMAScript,ReactandReactNative.However,youcansuccessfullytransferalotoftheskillsandtechniquestootherlanguages.Icallthem"Ideapatterns".ThisbookwillstartwiththemoststandarddevelopmentpatternsinReactlikecomponentbuildingpatterns,stylingpatternsinReactNativeandthenextendthesepatternstoyourmobileapplicationusingrealworldpracticalexamples.Eachchaptercomeswithfull,separatesourcecodeofapplicationsthatyoucanbuildandrunonyourphone.Thebookisalsodivingintoarchitecturalpatterns.EspeciallyhowtoadaptMVCtoReactenvironment.YouwilllearnFluxarchitectureandhowReduxisimplementingit.Eachapproachwillbepresentedwithitsprosandcons.YouwilllearnhowtoworkwithexternaldatasourcesusinglibrarieslikeReduxthunkandReduxSaga.Theendgoalistheabilitytorecognizethebestsolutionforagivenproblemforyournextmobileapplication.
目錄(233章)
倒序
- 封面
- Title Page
- Copyright and Credits
- Hands-On Design Patterns with React Native
- Dedication
- Packt.com
- Why subscribe?
- Packt.com
- Contributors
- About the author
- About the reviewer
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Reviews
- React Component Patterns
- Stateless and stateful components
- What are the advantages of stateless components?
- Component composition
- Composing the application layout
- What about component inheritance?
- Testing components on high-level patterns
- Snapshot testing expandable components
- Test-driven development approach
- Presentational components
- Decoupling styles
- Container component
- HOC
- HOC composition
- Examples of useful HOCs
- Summary
- View Patterns
- Technical requirements
- Introduction to JSX
- JSX standard tricks
- A beginner's guide to naming
- Type checking with PropTypes
- Built-in components you need to know about
- The ScrollView component
- The Image component
- The TextInput component
- The Button component
- Touchable opacity
- Building forms
- Controlled inputs
- Uncontrolled input
- Introduction to error boundaries
- How error boundaries catch errors
- Understanding error boundaries
- When to use error boundaries
- Why Mixins are anti-patterns
- Mixin example
- Using HOCs instead
- Linters and code style guide
- Adding a linter to create a React Native app
- Airbnb React style guide rules
- Fixing errors
- Summary
- Styling Patterns
- Technical requirements
- How React Native styles work
- Surprising styles inheritance
- Workaround for limited inheritance
- Learning unitless dimensions
- Absolute and relative positioning
- Using the Flexible Box pattern
- Positioning items with Flexbox
- Styling flex items
- Styling content
- Solving the text overflow problem
- Scaling the font down
- Truncating text
- Using the Kilo social media notation
- React Native animated
- What are animations?
- Changing attributes over time
- The easing function
- Scheduling events
- Measuring FPS
- How to measure FPS
- Summary
- Flux Architecture
- One-direction dataflow pattern
- React's one-way data binding
- Event problems
- Further issues with binding
- Introduction to Flux
- Replacing MVC
- Flux by example
- Detailed Flux diagram
- What are side effects?
- Why recognize side effects?
- Working with side effects in MVC
- Working with side effects in Flux
- Summary
- Questions
- Further reading
- Store Patterns
- Using Redux stores
- Minimal Redux application example
- How Redux fits into Flux
- Moving to Redux
- Redux as a pattern
- Core principles of Redux
- Moving to a single source of truth
- Creating an alternative with MobX
- Moving to MobX
- Using PropTypes with annotations
- Comparing Redux and MobX
- Using system storage in React Native
- Effect patterns
- Handling side effects
- Summary
- Further reading
- Data Transfer Patterns
- Preparation
- Fetching data with the built-in function
- Refactoring to activity indicator
- Handling error scenarios
- Naive stateful component fetching
- The Thunk pattern and Redux Thunk
- Lifting the state to Redux
- Benefits of refactoring to Redux
- Using Redux Thunk
- Understanding the Thunk pattern
- The saga pattern and Redux Saga
- Introduction to the iterator pattern
- The generator pattern
- Redux Saga
- Redux Saga benefits
- Summary
- Further reading
- Navigation Patterns
- React Native navigation alternatives
- Designers navigation patterns
- Navigation to top-level screens
- Navigating between different levels of the graph
- Navigating on the same level of the graph
- Developers' navigation patterns
- Restructuring your application
- React Navigation
- Using React Navigation
- Multiple screens with React Navigation
- Tab navigation
- Drawer navigation
- Issues with duplicated data
- React Native Navigation
- A few words on the setup
- Basics of React Native Navigation
- Further investigation
- Summary
- Further reading
- JavaScript and ECMAScript Patterns
- JavaScript and functional programming
- ES6 map filter and reduce
- Using reduce to reimplement filter and map
- Counting items in an array
- The iterator pattern
- Defining a custom iterator
- Using generators as a factory for iterators
- Making an API call to fetch task details with a generator
- Alternatives to generators
- Selectors
- Selecting from the Redux store
- Caching the selectors
- Learning functions from the Ramda library
- Composing functions
- Fighting the confusing code
- Currying functions
- Flipping
- Summary
- Further reading
- Elements of Functional Programming Patterns
- Mutable and immutable objects
- Immutable primitives in JavaScript
- Immutability cost explained
- Benchmark on read/write operations
- Pure functions
- Pure functions in Redux
- Caching pure functions
- Referential transparency
- Everything but monads
- Call me Maybe
- Monad interface requirements
- Higher-order functions
- Examples of higher-order functions
- Functional languages aside
- Terminology
- Building abstractions
- React is not obsessed with pure functions
- Summary
- Further reading
- Managing Dependencies
- The singleton pattern
- Implementing the singleton pattern in ECMAScript
- Why using the singleton pattern is discouraged
- The many singleton flavors in JavaScript
- ES6 modules and beyond
- The DI pattern
- Using the DI pattern with storybooks
- Nested stories with DI
- DI with React context
- Using the React Context API
- React Redux aside
- Managing the code base
- Quick wins
- Establishing conventions
- Summary
- Further reading
- Type Checking Patterns
- Introduction to types
- Introduction to TypeScript
- Configuring TypeScript
- Learning the basic types
- enums and constants patterns
- Creating union types and intersections
- Generic types
- Understanding TypeScript
- Type inference
- Structural typing
- Immutability with TypeScript
- readonly
- Using linter to enforce immutability
- Summary
- Further reading
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-08-13 15:13:36
推薦閱讀
- 軟件界面交互設計基礎
- Java入門很輕松(微課超值版)
- 神經網絡編程實戰:Java語言實現(原書第2版)
- 趣學Python算法100例
- Production Ready OpenStack:Recipes for Successful Environments
- Elasticsearch for Hadoop
- Android Wear Projects
- CoffeeScript Application Development Cookbook
- 細說Python編程:從入門到科學計算
- 交互式程序設計(第2版)
- Practical GIS
- Java設計模式深入研究
- Laravel Design Patterns and Best Practices
- Java從入門到精通(視頻實戰版)
- Clojure編程樂趣
- Mastering Python
- React and React Native
- Zend Framework 2 Cookbook
- 新手學ASP.NET 3.5網絡開發
- 編程真好玩:從零開始學網頁設計及3D編程
- OpenCV:Computer Vision Projects with Python
- Drupal 8 Module Development
- Developing Windows Store Apps with HTML5 and JavaScript
- Large Scale Machine Learning with Spark
- Android Studio 2 Essentials(Second Edition)
- Mastering Python Forensics
- AutoCAD 2008輔助設計
- PyTorch Computer Vision Cookbook
- 數據庫應用基礎(Access 2010)
- JavaEE主流開源框架