舉報
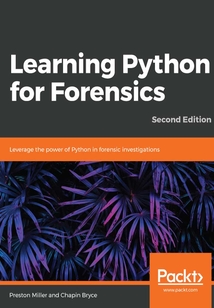
會員
Learning Python for Forensics
Digitalforensicsplaysanintegralroleinsolvingcomplexcybercrimesandhelpingorganizationsmakesenseofcybersecurityincidents.ThissecondeditionofLearningPythonforForensicsillustrateshowPythoncanbeusedtosupportthesedigitalinvestigationsandpermitstheexaminertoautomatetheparsingofforensicartifactstospendmoretimeexaminingactionabledata.ThesecondeditionofLearningPythonforForensicswillillustratehowtodevelopPythonscriptsusinganiterativedesign.Further,itdemonstrateshowtoleveragethevariousbuilt-inandcommunity-sourcedforensicsscriptsandlibrariesavailableforPythontoday.Thisbookwillhelpstrengthenyouranalysisskillsandefficiencyasyoucreativelysolvereal-worldproblemsthroughinstruction-basedtutorials.Bytheendofthisbook,youwillbuildacollectionofPythonscriptscapableofinvestigatinganarrayofforensicartifactsandmastertheskillsofextractingmetadataandparsingcomplexdatastructuresintoactionablereports.Mostimportantly,youwillhavedevelopedafoundationuponwhichtobuildasyoucontinuetolearnPythonandenhanceyourefficacyasaninvestigator.
目錄(327章)
倒序
- coverpage
- Title Page
- Copyright and Credits
- Learning Python for Forensics Second Edition
- About Packt
- Why subscribe?
- Packt.com
- Contributors
- About the authors
- About the reviewer
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Reviews
- Now for Something Completely Different
- When to use Python
- Development life cycle
- Getting started
- The omnipresent print() function
- Standard data types
- Strings and Unicode
- Integers and floats
- Boolean and none
- Structured data types
- Lists
- Dictionaries
- Sets and tuples
- Data type conversions
- Files
- Variables
- Understanding scripting flow logic
- Conditionals
- Loops
- The for loop
- The while loop
- Functions
- Summary
- Python Fundamentals
- Advanced data types and functions
- Iterators
- datetime objects
- Libraries
- Installing third-party libraries
- Libraries in this book
- Python packages
- Classes and object-oriented programming
- Try and except
- The raise function
- Creating our first script – unix_converter.py
- User input
- Using the raw input method and the system module – user_input.py
- Understanding Argparse – argument_parser.py
- Forensic scripting best practices
- Developing our first forensic script – usb_lookup.py
- Understanding the main() function
- Interpreting the search_key() function
- Running our first forensic script
- Troubleshooting
- Challenge
- Summary
- Parsing Text Files
- Setup API
- Introducing our script
- Overview
- Our first iteration – setupapi_parser_v1.py
- Designing the main() function
- Crafting the parse_setupapi() function
- Developing the print_output() function
- Running the script
- Our second iteration – setupapi_parser_v2.py
- Improving the main() function
- Tuning the parse_setupapi() function
- Modifying the print_output() function
- Running the script
- Our final iteration – setupapi_parser.py
- Extending the main() function
- Adding to the parse_setup_api() function
- Creating the parse_device_info() function
- Forming the prep_usb_lookup() function
- Constructing the get_device_names() function
- Enhancing the print_output() function
- Running the script
- Challenge
- Summary
- Working with Serialized Data Structures
- Serialized data structures
- A simple Bitcoin web API
- Our first iteration – bitcoin_address_lookup.v1.py
- Exploring the main() function
- Understanding the get_address() function
- Working with the print_transactions() function
- The print_header() helper function
- The get_inputs() helper function
- Running the script
- Our second iteration – bitcoin_address_lookup.v2.py
- Modifying the main() function
- Improving the get_address() function
- Elaborating on the print_transactions() function
- Running the script
- Mastering our final iteration – bitcoin_address_lookup.py
- Enhancing the parse_transactions() function
- Developing the csv_writer() function
- Running the script
- Challenge
- Summary
- Databases in Python
- An overview of databases
- Using SQLite3
- Using SQL
- Designing our script
- Manually manipulating databases with Python – file_lister.py
- Building the main() function
- Initializing the database with the init_db() function
- Checking for custodians with the get_or_add_custodian() function
- Retrieving custodians with the get_custodian() function
- Understanding the ingest_directory() function
- Exploring the os.stat() method
- Developing the format_timestamp() helper function
- Configuring the write_output() function
- Designing the write_csv() function
- Composing the write_html() function
- Running the script
- Automating databases further – file_lister_peewee.py
- Peewee setup
- Jinja2 setup
- Updating the main() function
- Modifying the get_or_add_custodian() function
- Improving the ingest_directory() function
- A closer look at the format_timestamp() function
- Converting the write_output() function
- Simplifying the write_csv() function
- Condensing the write_html() function
- Running our new and improved script
- Challenge
- Summary
- Extracting Artifacts from Binary Files
- UserAssist
- Understanding the ROT-13 substitution cipher – rot13.py
- Evaluating code with timeit
- Working with the yarp library
- Introducing the struct module
- Creating spreadsheets with the xlsxwriter module
- Adding data to a spreadsheet
- Building a table
- Creating charts with Python
- The UserAssist framework
- Developing our UserAssist logic processor – userassist_parser.py
- Evaluating the main() function
- Defining the create_dictionary() function
- Extracting data with the parse_values() function
- Processing strings with the get_name() function
- Writing Excel spreadsheets – xlsx_writer.py
- Controlling output with the excel_writer() function
- Summarizing data with the dashboard_writer() function
- Writing artifacts in the userassist_writer() function
- Defining the file_time() function
- Processing integers with the sort_by_count() function
- Processing datetime objects with the sort_by_date() function
- Writing generic spreadsheets – csv_writer.py
- Understanding the csv_writer() function
- Running the UserAssist framework
- Challenge
- Summary
- Fuzzy Hashing
- Background on hashing
- Hashing files in Python
- Hashing large files – hashing_example.py
- Creating fuzzy hashes
- Context Triggered Piecewise Hashing (CTPH)
- Implementing fuzzy_hasher.py
- Starting with the main() function
- Creating our fuzzy hashes
- Generating our rolling hash
- Preparing signature generation
- Providing the output
- Running fuzzy_hasher.py
- Using ssdeep in Python – ssdeep_python.py
- Revisiting the main() function
- Redesigning our output() function
- Running ssdeep_python.py
- Additional challenges
- References
- Summary
- The Media Age
- Creating frameworks in Python
- Introduction to EXIF metadata
- Introducing the Pillow module
- Introduction to ID3 metadata
- Introducing the Mutagen module
- Introduction to Office metadata
- Introducing the lxml module
- The Metadata_Parser framework overview
- Our main framework controller – metadata_parser.py
- Controlling our framework with the main() function
- Parsing EXIF metadata – exif_parser.py
- Understanding the exif_parser() function
- Developing the get_tags() function
- Adding the dms_to_decimal() function
- Parsing ID3 metdata – id3_parser.py
- Understanding the id3_parser() function
- Revisiting the get_tags() function
- Parsing Office metadata – office_parser.py
- Evaluating the office_parser() function
- The get_tags() function for the last time
- Moving on to our writers
- Writing spreadsheets – csv_writer.py
- Plotting GPS data with Google Earth – kml_writer.py
- Supporting our framework with processors
- Creating framework-wide utility functions – utility.py
- Framework summary
- Additional challenges
- Summary
- Uncovering Time
- About timestamps
- What's an epoch?
- Using a GUI
- Basics of TkInter objects
- Implementing the TkInter GUI
- Using frame objects
- Using classes in TkInter
- Developing the date decoder GUI – date_decoder.py
- The DateDecoder class setup and __init__() method
- Executing the run() method
- Implementing the build_input_frame() method
- Creating the build_output_frame() method
- Building the convert() method
- Defining the convert_unix_seconds() method
- Conversion using the convert_win_filetime_64() method
- Converting with the convert_chrome_time() method
- Designing the output method
- Running the script
- Additional challenges
- Summary
- Rapidly Triaging Systems
- Understanding the value of system information
- Querying OS-agnostic process information with psutil
- Using WMI
- What does the pywin32 module do?
- Rapidly triaging systems – pysysinfo.py
- Understanding the get_process_info() function
- Learning about the get_pid_details() function
- Extracting process connection properties with the read_proc_connections() function
- Obtaining more process information with the read_proc_files() function
- Extracting Windows system information with the wmi_info() function
- Writing our results with the csv_writer() function
- Executing pysysinfo.py
- Challenges
- Summary
- Parsing Outlook PST Containers
- The PST file format
- An introduction to libpff
- How to install libpff and pypff
- Exploring PSTs – pst_indexer.py
- An overview
- Developing the main() function
- Evaluating the make_path() helper function
- Iteration with the folder_traverse() function
- Identifying messages with the check_for_msgs() function
- Processing messages in the process_msg() function
- Summarizing data in the folder_report() function
- Understanding the word_stats() function
- Creating the word_report() function
- Building the sender_report() function
- Refining the heat map with the date_report() function
- Writing the html_report() function
- The HTML template
- Running the script
- Additional challenges
- Summary
- Recovering Transient Database Records
- SQLite WAL files
- WAL format and technical specifications
- The WAL header
- The WAL frame
- The WAL cell and varints
- Manipulating large objects in Python
- Regular expressions in Python
- TQDM – a simpler progress bar
- Parsing WAL files – wal_crawler.py
- Understanding the main() function
- Developing the frame_parser() function
- Processing cells with the cell_parser() function
- Writing the dict_helper() function
- The Python debugger – pdb
- Processing varints with the single_varint() function
- Processing varints with the multi_varint() function
- Converting serial types with the type_helper() function
- Writing output with the csv_writer() function
- Using regular expression in the regular_search() function
- Executing wal_crawler.py
- Challenge
- Summary
- Coming Full Circle
- Frameworks
- Building a framework to last
- Data standardization
- Forensic frameworks
- Colorama
- FIGlet
- Exploring the framework – framework.py
- Exploring the Framework object
- Understanding the Framework __init__() constructor
- Creating the Framework run() method
- Iterating through files with the Framework _list_files() method
- Developing the Framework _run_plugins() method
- Exploring the Plugin object
- Understanding the Plugin __init__() constructor
- Working with the Plugin run() method
- Handling output with the Plugin write() method
- Exploring the Writer object
- Understanding the Writer __init__() constructor
- Understanding the Writer run() method
- Our Final CSV writer – csv_writer.py
- The writer – xlsx_writer.py
- Changes made to plugins
- Executing the framework
- Additional challenges
- Summary
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時間:2021-08-20 10:17:57
推薦閱讀
- Kali Linux Social Engineering
- 黑客攻防入門秘笈
- 走進新安全:讀懂網絡安全威脅、技術與新思想
- Preventing Digital Extortion
- 代碼審計:企業級Web代碼安全架構
- 軟件開發安全之道:概念、設計與實施
- Kali Linux Wireless Penetration Testing Cookbook
- 解密彩虹團隊非凡實戰能力:企業安全體系建設(共5冊)
- API安全技術與實戰
- 網絡安全與攻防入門很輕松(實戰超值版)
- 互聯網企業安全高級指南
- Mastering Reverse Engineering
- 網絡安全大數據分析與實戰
- Bug Bounty Hunting Essentials
- 數字政府網絡安全合規性建設指南:密碼應用與數據安全
- 網絡空間安全導論
- Learn Azure Sentinel
- 大數據安全治理與防范:反欺詐體系建設
- 復雜網絡環境下訪問控制技術
- 商用密碼發展報告(2012—2017年)
- Securing Docker
- Implementing AppFog
- Practical PowerShell Exchange Server 2019
- 信息系統等級保護安全建設技術方案設計實現與應用
- 黑客攻防從入門到精通(社會工程學篇)
- 走近安全:網絡世界的攻與防
- 信息安全案例教程:技術與應用
- Becoming the Hacker
- 動手學差分隱私
- VMware vSphere Security Cookbook