舉報(bào)
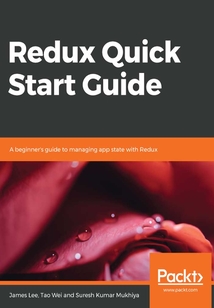
會員
Redux Quick Start Guide
StartingwithadetailedoverviewofRedux,wewillfollowthetest-drivendevelopment(TDD)approachtodevelopsingle-pageapplications.WewillsetupJESTfortestinganduseJESTtotestReact,Redux,Redux-Sage,Reducers,andothercomponents.WewillthenaddimportantmiddlewareandsetupimmutableJSinourapplication.WewillusecommondatastructuressuchasMap,List,Set,andOrderedListfromtheimmutableJSframework.WewillthenadduserinterfacesusingReactJS,Redux-Form,andAntDesign.Wewillexploretheuseofreact-router-domanditsfunctions.Wewillcreatealistofroutesthatwewillneedinordertocreateourapplication,andexploreroutingontheserversiteandcreatetherequiredroutesforourapplication.WewillthendebugourapplicationandintegrateReduxDevtools.WewillthensetupourAPIserverandcreatetheAPIrequiredforourapplication.WewilldiveintoamodernapproachtostructuringourserversitecomponentsintermsofModel,Controller,Helperfunctions,andutilitiesfunctions.WewillexploretheuseofNodeJSwithExpresstobuildtheRESTAPIcomponents.Finally,wewillventureintothepossibilitiesofextendingtheapplicationforfurtherresearch,includingdeploymentandoptimization.
目錄(178章)
倒序
- coverpage
- Title Page
- Copyright and Credits
- Redux Quick Start Guide
- Dedication
- About Packt
- Why subscribe?
- Packt.com
- Contributors
- About the authors
- About the reviewers
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Download the example code files
- Conventions used
- Get in touch
- Reviews
- Understanding Redux
- The need for Redux
- Functional programming
- Assigning functions to variables
- Adding functions to objects and arrays
- Functions as arguments
- Functions returned by functions
- Higher-order functions
- Pure functions
- Compositions
- Fundamental principles of Redux
- Single source of truth
- Read-only nature of the state
- The reducer principle – changes are made with pure functions
- The Redux ecosystem
- Elements of Redux
- Actions
- Action creators
- Reducers
- Store
- getState()
- dispatch(action)
- subscribe(listeners)
- replaceReducer(nextReducer)
- Redux life cycle
- Getting started
- Understanding Redux methods
- Setting up the project
- Configuring the store
- Configuring the root reducer
- Configuring our app with Redux
- Creating utilities
- Creating the first container
- Summary
- Further study
- Testing
- Setting up Jest
- Testing ES6 functions
- Testing a function
- A general testing scenario
- Time mocks
- Testing React components
- React components and mocking components
- Multiple React components
- Testing event handlers
- Testing Redux
- Testing action creators
- Testing reducers
- Higher-order functions
- Summary
- Further reading
- Routing
- Using react-router-dom
- Understanding route props
- The Redirect component
- Using connected-react-router
- History
- Mocking react-router-dom for testing
- Summary
- Further reading
- The Concept of Immutability
- The need for immutability
- The data reference problem
- Reference handling
- Getting started with Immutable JS
- Components of Immutable JS
- FromJS
- Map
- List
- Set
- The TODO app
- Components
- Reducers
- Connecting with Redux
- Using Immutable JS in our project
- The Immutable JS ecosystem
- Frequently asked questions
- Summary
- Further reading
- React with Redux
- Components of React
- Principles of React
- New in React 16.8
- New features with React 16.8
- User interfaces
- Project structure
- React component libraries
- Antd
- styled-component
- Redux form
- containers/App/index.js
- Login page
- Register page
- Users pages
- Listing all of the users
- Adding a new user page
- Connecting with Redux
- Action types
- Connecting with Redux
- Connecting the login page with Redux
- Action creators
- Constant
- Connect
- Connecting an add user page to Redux
- Login page reducer
- Understanding selectors
- Why selectors?
- reselect
- Summary
- Further study
- Extending Redux with Middleware
- Exploring middleware
- Router middleware
- redux-saga middleware
- Getting started
- Adding Saga to the application
- Connecting the Saga middleware to the store
- Using the REST API
- Prerequisites
- Seeding users
- Seeding doctors
- Seeding admin
- Connecting the login functionality with the API
- Creating Saga
- Passing the subset of the state to a component
- Connecting the home page with the API
- CRUD on users
- Defining Saga
- Language middleware
- Summary
- Further study
- Debugging Redux
- Integrating Redux DevTools
- Installing Redux DevTools
- Redux DevTools extension
- Understanding Redux DevToolsHMR
- Replacing the hot module
- Loading the hot module
- Summary
- Further reading
- Understanding the REST API
- The REST principle
- The HTTP verbs and HTTP response status code
- Project structure
- Seeding users
- User endpoints
- POST – Creating a user
- GET – List of users
- Authentication and authorization
- Authentication
- Authorization
- Getting a single user information
- Updating user information
- Other endpoints
- Summary
- Further reading
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時(shí)間:2021-07-02 12:40:54
推薦閱讀
- Mastering Concurrency Programming with Java 8
- 手機(jī)安全和可信應(yīng)用開發(fā)指南:TrustZone與OP-TEE技術(shù)詳解
- Docker and Kubernetes for Java Developers
- Learning Cython Programming
- 案例式C語言程序設(shè)計(jì)
- C程序設(shè)計(jì)簡明教程(第二版)
- Mastering JavaScript Object-Oriented Programming
- ASP.NET Core Essentials
- Java程序設(shè)計(jì)與計(jì)算思維
- Visual C++數(shù)字圖像處理技術(shù)詳解
- Mastering Ext JS
- 動手學(xué)數(shù)據(jù)結(jié)構(gòu)與算法
- Learning Splunk Web Framework
- 大規(guī)模語言模型開發(fā)基礎(chǔ)與實(shí)踐
- 數(shù)字媒體技術(shù)概論
- 軟件設(shè)計(jì)模式(Java版)
- 軟件測試項(xiàng)目實(shí)戰(zhàn)之功能測試篇
- Unity與C++網(wǎng)絡(luò)游戲開發(fā)實(shí)戰(zhàn):基于VR、AI與分布式架構(gòu)
- Visual Basic.NET程序設(shè)計(jì)
- R語言編程:基于tidyverse
- 計(jì)算機(jī)網(wǎng)絡(luò)基礎(chǔ)
- 中文版AutoCAD 2017實(shí)用教程
- Three.js開發(fā)指南
- 學(xué)習(xí)JavaScript數(shù)據(jù)結(jié)構(gòu)與算法(第3版)
- 麥克奇遇記:Scratch 2.0探險(xiǎn)之旅(創(chuàng)客教育)
- Kali Linux Wireless Penetration Testing:Beginner's Guide
- Microsoft BizTalk ESB Toolkit 2.1
- 深入理解Java虛擬機(jī):JVM高級特性與最佳實(shí)踐(第2版)
- Java多線程編程核心技術(shù)(第3版)
- Android開發(fā)寶典