首頁 > 計(jì)算機(jī)網(wǎng)絡(luò) >
編程語言與程序設(shè)計(jì)
> Mastering React Test:Driven Development最新章節(jié)目錄
舉報(bào)
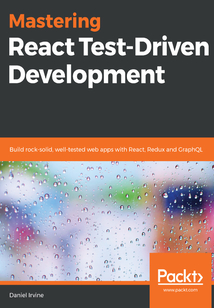
會(huì)員
Mastering React Test:Driven Development
ManyprogrammersareawareofTDDbutstruggletoapplyitbeyondbasicexamples.Thisbookteacheshowtobuildcomplex,real-worldapplicationsusingTest-DrivenDevelopment(TDD).IttakesafirstprinciplesapproachtotheTDDprocessusingplainJestandincludestest-drivingtheintegrationoflibrariesincludingReactRouter,Redux,andRelay(GraphQL).Readerswillpracticesystematicrefactoringwhilebuildingouttheirowntestframework,gainingadeepunderstandingofTDDtoolsandtechniques.Theywilllearnhowtotest-drivefeaturessuchasclient-andserver-sideformvalidation,datafilteringandsearching,navigationanduserworkflow,undo/redo,animation,LocalStorageaccess,WebSocketcommunication,andqueryingGraphQLendpoints.ThebookcoversrefactoringcodebasestousetheReactRouterandReduxlibraries.viaTDD.Reduxisexploredindepth,withreducers,middleware,sagas,andconnectedReactcomponents.ThebookalsocoversacceptancetestingusingCucumberandPuppeteer.ThebookisfullyuptodatewithReact16.9andhasin-depthcoverageofhooksandthe‘a(chǎn)ct’testhelper.
目錄(285章)
倒序
- coverpage
- Title Page
- Copyright and Credits
- Mastering React Test-Driven Development
- Dedication
- About Packt
- Why subscribe?
- Packt.com
- Contributors
- About the author
- About the reviewer
- Packt is searching for authors like you
- Preface
- Who this book is for
- What this book covers
- To get the most out of this book
- Keeping up with the book's Git history
- Getting started before Chapter 1
- Working with section tags
- Solving the exercises
- Debugging when things go wrong
- Download the example code files
- Conventions used
- Understanding code snippets
- JavaScript syntax
- Prettier
- Arrow functions
- Object and array destructuring
- Directory structure
- Get in touch
- Reviews
- Section 1: First Principles of TDD
- First Steps with Test-Driven Development
- Technical requirements
- Creating a new React project from scratch
- Installing NPM
- Creating a new Jest project
- Commit early and often
- Bringing in React and Babel
- Displaying data with your first test
- Writing a failing test
- Writing your first expectation
- Rendering React from a test
- Make it pass
- Backtracking on ourselves
- Refactoring your work
- Promoting variables
- Using a beforeEach block
- Extracting methods
- Writing great tests
- Red green refactor
- Streamlining your testing process
- Rendering lists and detail views
- Rendering the list of appointments
- Specifying list items
- Selecting data to view
- Initial selection of data
- Adding events to a functional component
- Manually testing our changes
- Adding an entrypoint
- Putting it all together with Webpack
- Before you check in...
- Summary
- Exercises
- Further learning
- Test-driving Data Input with React
- Extracting a test helper
- Adding a form element
- Extracting a form-finder method
- Accepting text input
- Extracting an expectation group function
- Passing in an existing value
- Extracting out a field-finder function
- Labeling the field
- Checking for null or not
- Saving the customer information
- Submitting a form with data
- Using state instead of props
- Duplicating fields
- Nesting describe blocks
- Generating parameterized tests
- Solving a batch of tests
- Modifying handleChange to work with multiple fields
- Finishing off the form with a submit button
- Selecting from a dropdown
- Providing options to a dropdown
- Utilizing defaultProps to specify real data
- Pre-selecting a value
- Completing the remaining tests for the select box
- Making a choice from radio buttons
- Constructing a calendar view
- Displaying radio buttons for available appointments
- Hiding input controls
- Finishing it off
- Manually testing your solution
- Summary
- Exercises
- Further learning
- Exploring Test Doubles
- What is a test double?
- Learning to avoid fakes
- Submitting forms using spies
- Untangling Arrange-Act-Assert
- Watching it fail
- Making spies reusable
- Using a Jest matcher to simplify expectations
- Stubbing the fetch API
- Replacing global variables with spies
- Installing the window.fetch polyfill
- Acting on return values with stubs
- Acting on the fetch response
- Displaying errors to the user
- Extracting test helpers
- Using Jest to spy and stub
- Extracting spy helpers
- Using jest.spyOn to spy on module mocks
- Drying up DOM queries
- Extracting container.querySelectorAll
- Drying up DOM events
- Summary
- Exercises
- Further learning
- Creating a User Interface
- Fetching data on load with useEffect
- Stubbing exported constants
- Using props within useEffect
- Passing customer data through to AppointmentForm
- Passing through props to the child component
- Working with the shallow renderer
- Understanding the importance of spiking
- Building shallow renderer helpers
- Listing element children
- Encapsulating render output to dry up tests
- Building a new root component
- Summary
- Further learning
- Section 2: Building a Single-Page Application
- Humanizing Forms
- Performing client-side validation
- Submitting the form
- Extracting non-React functionality into a new module
- Handling server errors
- Indicating that the form has been submitted
- Refactoring long methods
- Summary
- Exercises
- Further learning
- Filtering and Searching Data
- Displaying tabular data fetched from an endpoint
- Paging through a large data set
- Adding a next page button
- Adding a previous page button
- Filtering data
- Refactoring to simplify component design
- Adding table row actions
- Specifying the render prop in App
- Summary
- Exercises
- Test-driving React Router
- General rules for test-driving React Router
- Using shallow rendering for the simplest results
- Passing React Router props down through your components
- Avoiding withRouter
- Building a root component
- Using the Router Switch component
- Testing the default route
- Invoking render functions and inspecting their properties
- Changing location using history.push
- Using the location query string to store component state
- Replacing onClick handlers with Link components
- Using a parent component to convert a query string to props
- Replacing onChange handlers with history.push
- Summary
- Exercises
- Further learning
- Test-driving Redux
- Prerequisites
- Test-driving a Redux saga
- Designing the state object
- Scaffolding the saga and reducer
- Scaffolding a reducer
- Setting up an entrypoint
- Making asynchronous requests with sagas
- Completing the reducer
- Pulling out generator functions for reducer actions
- Switching out component state for Redux state
- Building a helper function to render with store
- Submitting a React form by dispatching a Redux action
- Protecting against silent breakages
- Shifting workflow to Redux
- Stubbing out components built with useMemo
- Navigating router history in a Redux saga
- Separating Redux connection from presentation
- Summary
- Exercises
- Further learning
- Test-driving GraphQL
- Installing Relay
- Testing the Relay environment
- Building the GraphQL reducer
- Building the CustomerHistory component
- Tying it together in App
- Compiling Relay queries
- Summary
- Exercises
- Further learning
- Section 3: Interactivity
- Building a Logo Interpreter
- Studying the Spec Logo user interface
- Looking through the codebase
- Undoing and redoing user actions in Redux
- Building the reducer
- Setting the initial state
- Handling the undo action
- Handling the redo action
- Attaching the new reducer
- Building buttons
- Saving to LocalStorage via Redux middleware
- Building middleware
- Changing keyboard focus
- Writing the reducer
- Adding the reducer to the store
- Focusing the prompt
- Requesting focus in other components
- Summary
- Further learning
- Adding Animation
- Isolating components for animation
- Designing the component
- Extracting out StaticLines
- Building an AnimatedLine component
- Animating with requestAnimationFrame
- Drawing lines
- Cleaning up after useEffect
- Rotating the turtle
- Summary
- Exercises
- Working with WebSockets
- Designing a WebSocket interaction
- The new UI elements
- Splitting apart the saga
- Test-driving a WebSocket connection
- Streaming events with redux-saga
- Updating the app
- Summary
- Exercises
- Further learning
- Section 4: Acceptance Testing with BDD
- Writing Your First Acceptance Test
- Integrating Cucumber and Puppeteer into your code base
- Writing your first Cucumber test
- Using data tables to perform setup
- Summary
- Adding Features Guided by Acceptance Tests
- Adding acceptance tests for a dialog box
- Fixing acceptance tests by test-driving production code
- Adding a dialog box
- Updating sagas to reset or replay state
- Adding better wait support
- Alerting when the animation is complete
- Updating step definitions to use waitForSelector
- Exercises
- Summary
- Understanding TDD in the Wider Testing Landscape
- Test-driven development as a testing technique
- Best practices for your unit tests
- Improving your technique
- Manual testing
- Demonstrating software
- Testing the whole product
- Exploratory testing
- Debugging in the browser
- Automated testing
- Integration tests
- Acceptance tests
- Property-based and generative testing
- Snapshot testing
- Canary testing
- Not testing at all
- When quality doesn't matter
- Spiking and deleting code
- Summary
- Further learning
- Other Books You May Enjoy
- Leave a review - let other readers know what you think 更新時(shí)間:2021-06-24 14:45:49
推薦閱讀
- Web應(yīng)用系統(tǒng)開發(fā)實(shí)踐(C#)
- 復(fù)雜軟件設(shè)計(jì)之道:領(lǐng)域驅(qū)動(dòng)設(shè)計(jì)全面解析與實(shí)戰(zhàn)
- Java范例大全
- Python從小白到大牛
- 微服務(wù)設(shè)計(jì)原理與架構(gòu)
- Python機(jī)器學(xué)習(xí)編程與實(shí)戰(zhàn)
- FPGA Verilog開發(fā)實(shí)戰(zhàn)指南:基于Intel Cyclone IV(進(jìn)階篇)
- RSpec Essentials
- Mastering Business Intelligence with MicroStrategy
- ASP.NET程序開發(fā)范例寶典
- Java 從入門到項(xiàng)目實(shí)踐(超值版)
- Python Machine Learning Blueprints:Intuitive data projects you can relate to
- Practical GIS
- 深度實(shí)踐KVM:核心技術(shù)、管理運(yùn)維、性能優(yōu)化與項(xiàng)目實(shí)施
- Mastering ASP.NET Core 2.0
- AngularJS UI Development
- Java EE 7 Development with WildFly
- Python程序設(shè)計(jì):基礎(chǔ)與實(shí)踐
- Spring MVC Cookbook
- R語言編程:基于tidyverse
- Access 2013數(shù)據(jù)庫應(yīng)用案例課堂
- Java基礎(chǔ)案例教程
- Arduino Computer Vision Programming
- 性能之道:分布式系統(tǒng)全棧性能優(yōu)化
- Drupal 7 Media
- PostgreSQL Development Essentials
- Unity 5.x Shaders and Effects Cookbook
- Dart By Example
- Java Web程序設(shè)計(jì)與案例教程(微課版)
- Programming Arduino with LabVIEW